Android Holo ColorPicker
Marie Schweiz http://marie-schweiz.de/ made a beautifull new design for the Holo ColorPicker which added a lot of new functionality.
You can now set the Saturation and Value of a color. Also its possible to set the Opacity for a color.
You can also set the last selected color and see the difference with the new selected color.
Demo can be found on my Google Drive here if interested. the code of the sample can be found at a gist here
Now bars can change their orientation, Thanks to [tonyr59h]( UDPATEhttps://github.com/tonyr59h) also the gradle build version was updated to 0.7.+ 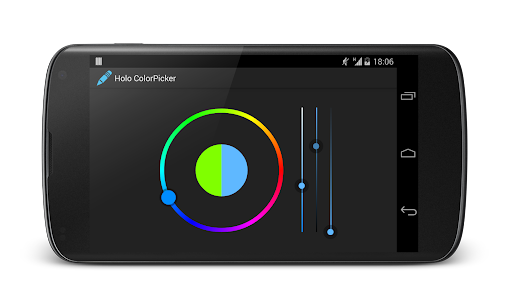
Documentation
To add the ColorPicker to your layout add this to your xml
<com.larswerkman.holocolorpicker.ColorPicker
android:id="@+id/picker"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
To add a Saturation/Value bar to your layout add this to your xml
<com.larswerkman.holocolorpicker.SVBar
android:id="@+id/svbar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
The same goes for the Opacity bar
<com.larswerkman.holocolorpicker.OpacityBar
android:id="@+id/opacitybar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
Saturation bar
<com.larswerkman.holocolorpicker.SaturationBar
android:id="@+id/saturationbar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
and a Value bar
<com.larswerkman.holocolorpicker.ValueBar
android:id="@+id/valuebar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
To connect the bars with the colorpicker and to get the selected color.
ColorPicker picker = (ColorPicker) findViewById(R.id.picker);
SVBar svBar = (SVBar) findViewById(R.id.svbar);
OpacityBar opacityBar = (OpacityBar) findViewById(R.id.opacitybar);
SaturationBar saturationBar = (SaturationBar) findViewById(R.id.saturationbar);
ValueBar valueBar = (ValueBar) findViewById(R.id.valuebar);
picker.addSVBar(svBar);
picker.addOpacityBar(opacityBar);
picker.addSaturationBar(saturationBar);
picker.addValueBar(valueBar);
//To get the color
picker.getColor();
//To set the old selected color u can do it like this
picker.setOldCenterColor(picker.getColor());
// adds listener to the colorpicker which is implemented
//in the activity
picker.setOnColorChangedListener(this);
//to turn of showing the old color
picker.setShowOldCenterColor(false);
//adding onChangeListeners to bars
opacitybar.setOnOpacityChangeListener(new OnOpacityChangeListener …)
valuebar.setOnValueChangeListener(new OnValueChangeListener …)
saturationBar.setOnSaturationChangeListener(new OnSaturationChangeListener …)
Adding it as a dependency to your project. Dependency
dependencies {
compile 'com.larswerkman:HoloColorPicker:1.5'
}
License
Copyright 2012 Lars Werkman
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.