Bug Report
What happened?
We tried to open PDF (LCP) file both in our app and test app. We were able to successfully open it. However, we experienced an empty Table of Contents . We tried the same file without the LCP, we were able to get the Table of Contents successfully.
mediaType : application/pdf+lcp
Extension: lcpdf
Sample PDF LCP
https://tuxedo-preprod-icdp.yondu.net/api/ebook/mobile/lcp/get-book-lcp/be7ec450-4617-4b53-bc92-d910951636ea/293
[passphrase: 293]
Screenshot of PDF with LCP with table of contents
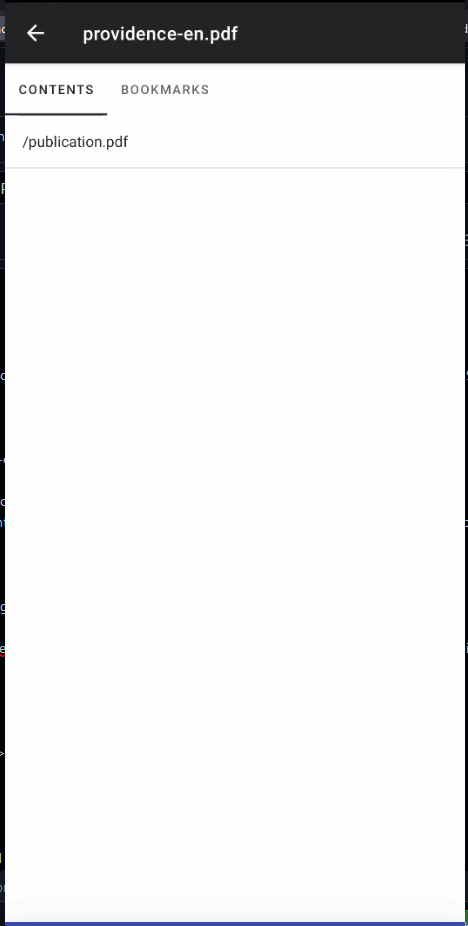
Sample PDF without LCP
https://document.desiringgod.org/providence-en.pdf?ts=1614698206
Screenshot of PDF without LCP with table of contents
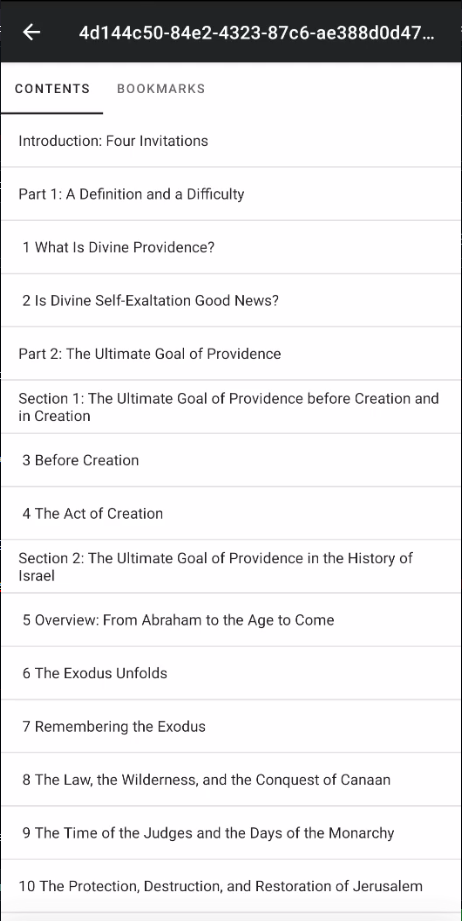
We tried to view the PDF with LCP in Thorium it can fetch the publication's table contents, however in the test app and Android app, we can't retrieve it. Hope you can assist us on how to fetch it thanks!
My teammate also notified me that this is also experienced in iOS as well.
Expected behavior
We expected to view the table of content list same with the PDF without the LCP.
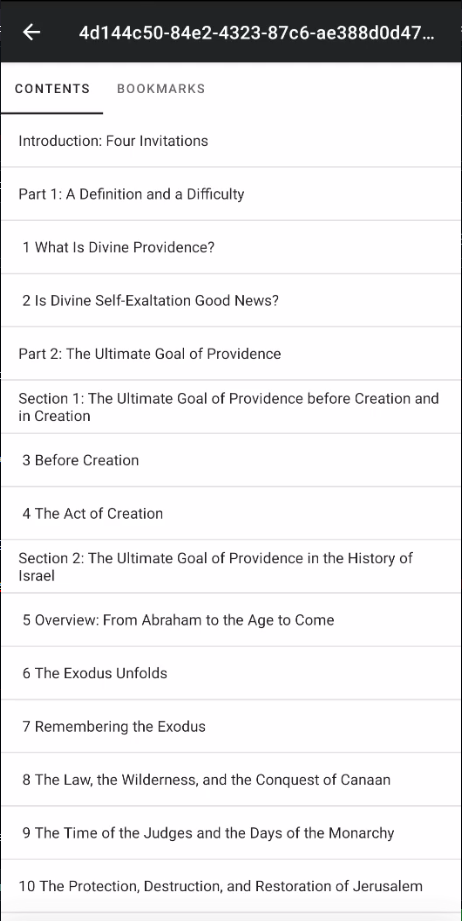
How to reproduce?
To test Sample PDF with LCP
- Press the floating "+" button and select Enter a URL.
- Enter https://tuxedo-preprod-icdp.yondu.net/api/ebook/mobile/lcp/get-book-lcp/be7ec450-4617-4b53-bc92-d910951636ea/293
- Enter 293 as passphrase.
- View Table of Contents (from the Option Hamburger menu).
To test Sample PDF without LCP
- Press the floating "+" button and select Enter a URL.
- Enter https://document.desiringgod.org/providence-en.pdf?ts=1614698206
- View Table of Contents (from the Option Hamburger menu).
Environment
- Readium version:
I'm not sure what the Readium version is. We imported the whole project from Github.
I tried to get the maven repository for this instead however we are experiencing error when integrating with LCP.
implementation "readium:liblcp:1.0.0@aar"
so we copy and pasted the whole library code instead.
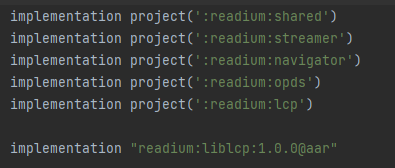
Development environment
Android Studio Chipmunk | 2021.2.1 Patch 1
Build #AI-212.5712.43.2112.8609683, built on May 19, 2022
Runtime version: 11.0.12+7-b1504.28-7817840 amd64
VM: OpenJDK 64-Bit Server VM by Oracle Corporation
Windows 10 10.0
GC: G1 Young Generation, G1 Old Generation
Memory: 1280M
Cores: 8
Registry: external.system.auto.import.disabled=true
Testing device
- Android version: 10
- Model: Huawei P20 Pro
- Is it an emulator? No, However we tested this in an emulator as well. We also tested this from other android devices too.
Additional context
- Are you willing to fix the problem and contribute a pull request? Yes or No
Sorry I can't right now.
But to be transparent, we updated the saving of Highlight DB's primary key to String (generate UUID) to be the same with iOS, so we can sync it with iOS.
Output logs
I added Logs to view Table of Content list in PdfReaderFragment (org.readium.r2.testapp.reader)
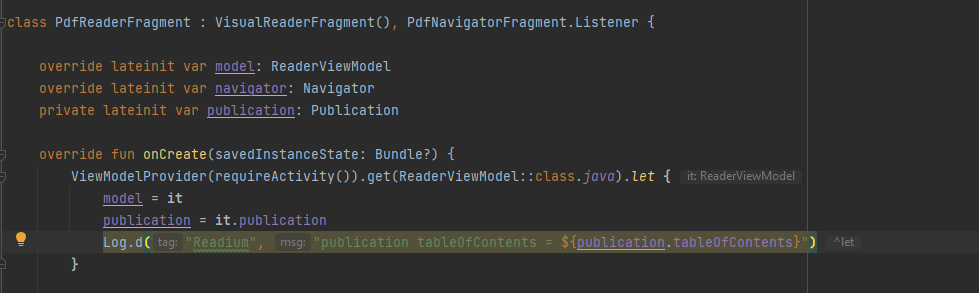
Sample PDF without LCP
2022-09-19 17:34:41.515 12617-12617/org.readium.r2reader D/Readium: publication tableOfContents = [Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=14, type=application/pdf, templated=false, title=Introduction: Four Invitations, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=28, type=application/pdf, templated=false, title=Part 1: A Definition and a Difficulty , rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=30, type=application/pdf, templated=false, title= 1 What Is Divine Providence?, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=40, type=application/pdf, templated=false, title= 2 Is Divine Self-Exaltation Good News?, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=48, type=application/pdf, templated=false, title=Part 2: The Ultimate Goal of Providence , rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=50, type=application/pdf, templated=false, title=Section 1: The Ultimate Goal of Providence before Creation and in Creation , rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=52, type=application/pdf, templated=false, title= 3 Before Creation, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=60, type=application/pdf, templated=false, title= 4 The Act of Creation, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=70, type=application/pdf, templated=false, title=Section 2: The Ultimate Goal of Providence in the History of Israel , rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=72, type=application/pdf, templated=false, title= 5 Overview: From Abraham to the Age to Come, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=88, type=application/pdf, templated=false, title= 6 The Exodus Unfolds, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=100, type=application/pdf, templated=false, title= 7 Remembering the Exodus, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=112, type=application/pdf, templated=false, title= 8 The Law, the Wilderness, and the Conquest of Canaan, rels=[], properties=Properties(otherProperties={}), height=null, width=null, bitrate=null, duration=null, languages=[], alternates=[], children=[]), Link(href=/4d144c50-84e2-4323-87c6-ae388d0d47c5.pdf#page=126, type=application/pdf, templated=false, title= 9 The Time of the Judges and the Days of the Monarchy, rels=[], propertie
Sample PDF LCP
2022-09-19 17:34:36.615 12617-12617/org.readium.r2reader D/Readium: publication tableOfContents = []
enhancement