Picasso Transformations
An Android transformation library providing a variety of image transformations for Picasso.
Please feel free to use this.
Are you using Glide or Fresco?
Glide Transformations
Fresco Processors
Demo
Original Image
Transformations
How do I use it?
Step 1
Gradle
repositories {
mavenCentral()
}
dependencies {
compile 'jp.wasabeef:picasso-transformations:2.4.0'
// If you want to use the GPU Filters
compile 'jp.co.cyberagent.android:gpuimage:2.1.0
}
Step 2
Set Picasso Transform.
Picasso.with(mContext).load(R.drawable.demo)
.transform(transformation).into((ImageView) findViewById(R.id.image));
Advanced Step 3
You can set a multiple transformations.
Picasso.with(mContext).load(R.drawable.demo)
.transform(transformation)
.transform(new CropCircleTransformation())
.into(holder.image);
Transformations
Crop
CropTransformation
, CropCircleTransformation
, CropSquareTransformation
, RoundedCornersTransformation
Color
ColorFilterTransformation
, GrayscaleTransformation
Blur
BlurTransformation
Mask
MaskTransformation
GPUImage)
GPU Filter (useWill require add dependencies for GPUImage.
ToonFilterTransformation
, SepiaFilterTransformation
, ContrastFilterTransformation
InvertFilterTransformation
, PixelationFilterTransformation
, SketchFilterTransformation
SwirlFilterTransformation
, BrightnessFilterTransformation
, KuwaharaFilterTransformation
VignetteFilterTransformation
Applications using Picasso Transformations
Please ping me or send a pull request if you would like to be added here.
Icon | Application |
---|---|
Ameba Ownd | |
QuitNow! |
Developed By
Daichi Furiya (Wasabeef) - [email protected]
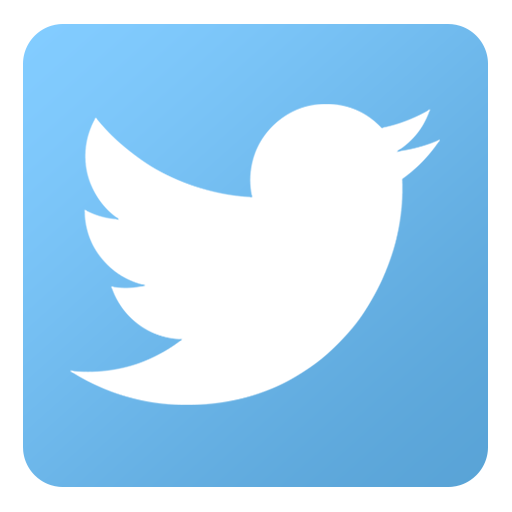
Thanks
- Inspired by
Picasso Transformations
in TannerPerrien.
License
Copyright (C) 2020 Wasabeef
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.