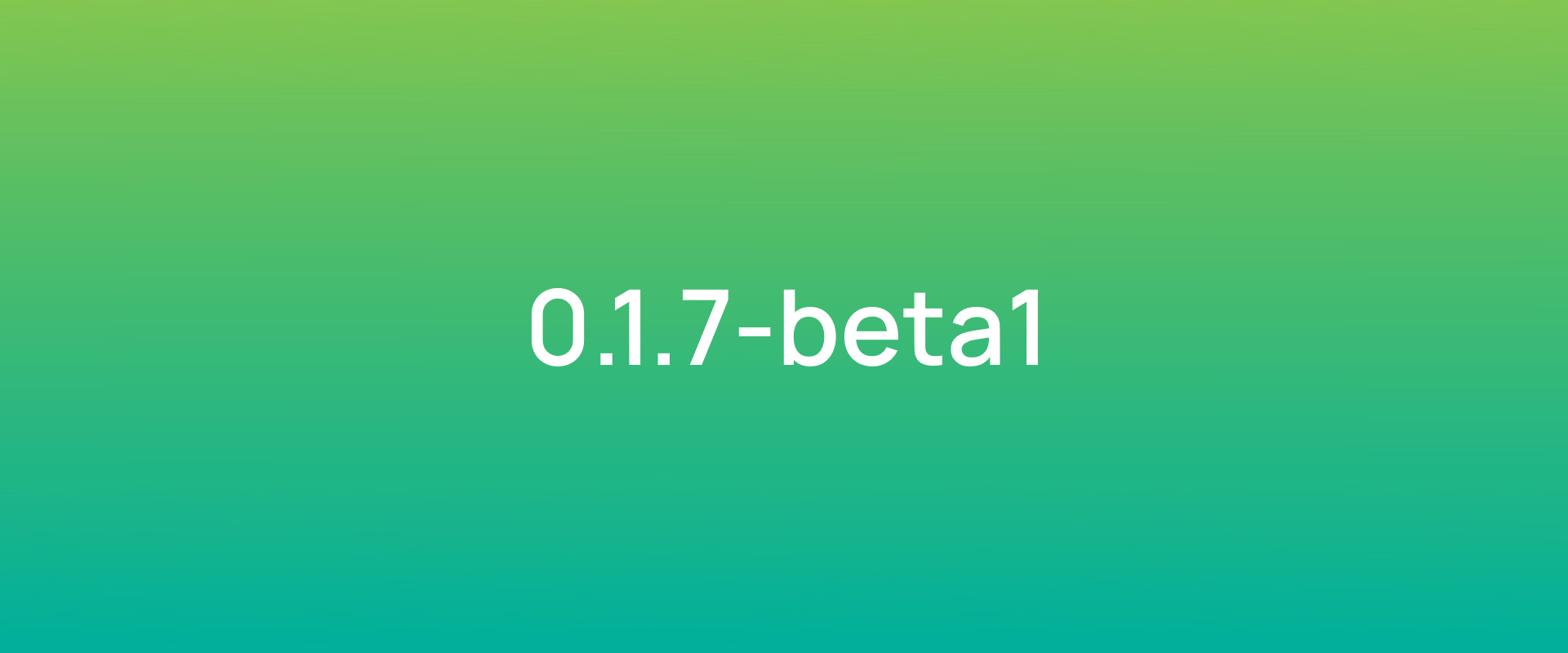
Before Updating, Read Carefully...
Since this is a beta version, expect bugs. For most users, it's worth waiting until 0.1.7.
The item_rotation
column has been removed from the database, permanently.
Rotation is still supported in the app, but the removal of this column has reduced unneeded complexity. I have provided a path for Room to ensure smooth database migration, although the only caveat is that after updating, all rotations done to your projects will reset. It's unfortunate that this occurs, although it shouldn't be a major issue, you can simply rotate the project again, press 'Save', and it will be back to what it was before. You may also experience further database bugs -- if I can find any more, I will fix them in 0.1.7.
I am looking for new collaborators
Apologies if this message bugs anyone, you can skip this if you're not interested.
PixaPencil is now open for serious contributors/collaborators who are interested in improving the app -- if you are interested in helping speed up the app's development process (and expand the app's reach) send me an email at therealbluepandabear@protonmail.com.
If you don't want to develop per se, but simply help test versions before they get released or help in documentation writing or cover art, that would also be great, and you can send me an email as well.
Features Added
✨ [BETA] Import external Lospec palettes as an in-app palette
Users now have the ability to import external Lospec palettes inside the app.
This feature is currently in beta, so it's not 100% stable.
More information
You can get to this by pressing the three dots at the top right of the screen, and then tapping 'Import Lospec Palette':
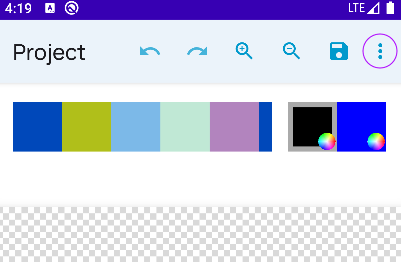
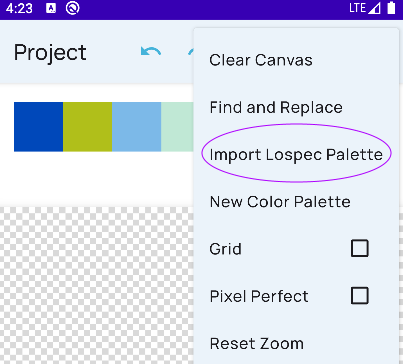
Then, type in the palette URL identifier of your choice:

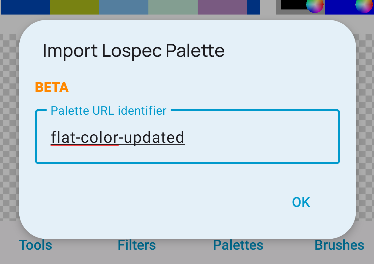
After pressing 'OK', the end result should be similar to the following:
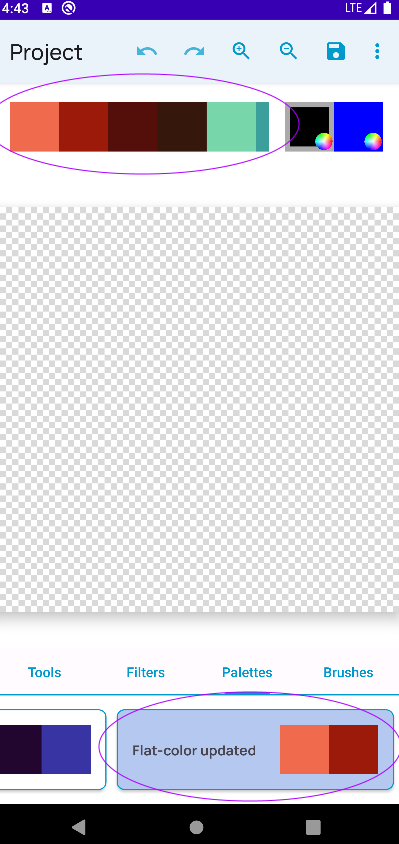
Improvements Added
- More strings have been translated into various languages -- thanks to all the translators!
- After 'Delete' is tapped in the bottom sheet, instead of getting a dialog verifying whether or not the user wants to delete their creation, the creation will get deleted and you will then see a snackbar with an 'Undo' button (20462bec3509eaee7300d9f5d7da109bc79938e8)
- Added a better ripple color for the floating action button in the home screen (8f5f26a84cee6fec1ab53a4e7c5c735044d36d85)
- 'Find and Replace', 'Filters', and 'Clear Canvas' actions are now faster (f5dcdcd9593252aec77bb921a9b478b7472a001f, 285b8bc2be519954d44bd4f7bc8099e33ce87b11, 62a13aa378b480f5c98856b2c8f68ba29669a552, ca48d41d5201d52769040c801838890332707b92) (#166)
Other Changes
- Backend improvements and general app stabilization
- Changelogs should now be visible on F-Droid (#156)
Source code(tar.gz)
Source code(zip)
app-debug.apk(13.50 MB)
app-release.apk(12.18 MB)