I'm using a TabLayout + ViewPager with two Fragments that should contain an AnyChart.
When the Fragment is created I call an Asynctask, that prepares the data for the AnyChart.
After the background-task the data should displayed in the AnyChart.
But i only get this:
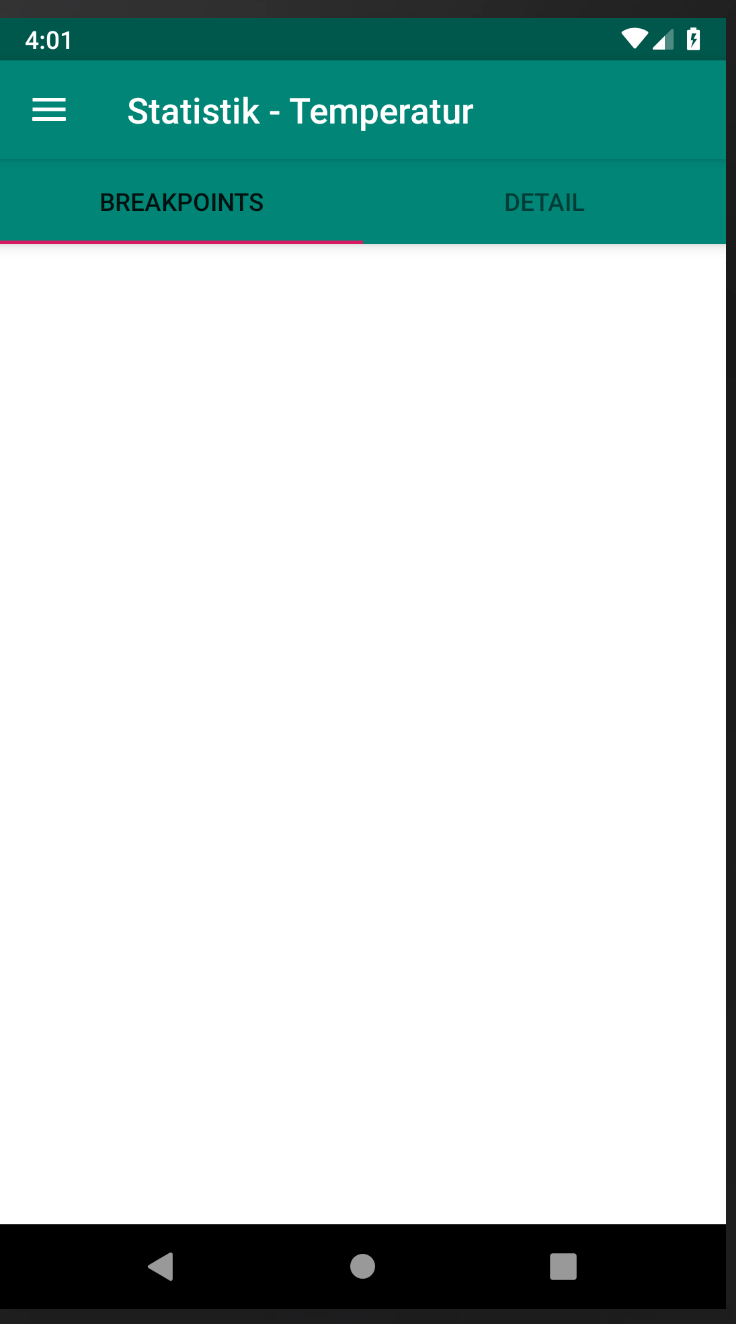
Instead of something like this:
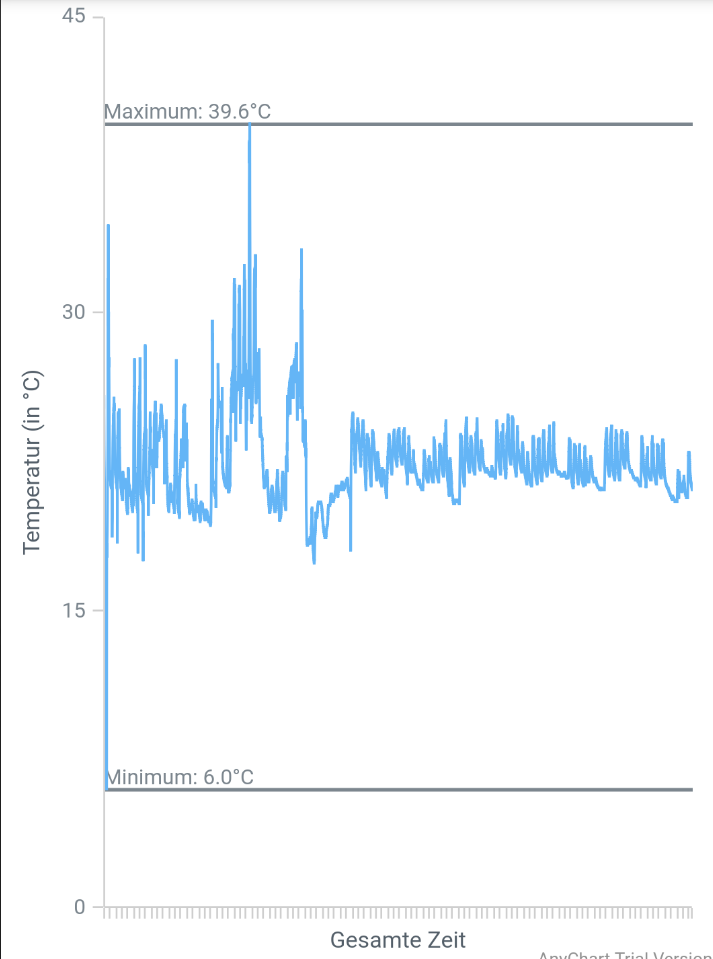
This are the TabLayout + ViewPager:
public class TemperatureStatisticsFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.statistics_temperature_fragment, container, false);
}
@Override
public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
Objects.requireNonNull(getActivity()).setTitle("Statistik - Temperatur");
TabLayout tabLayout = (TabLayout) getActivity().findViewById(R.id.tab_layout);
tabLayout.addTab(tabLayout.newTab().setText("Breakpoints"));
tabLayout.addTab(tabLayout.newTab().setText("Detail"));
tabLayout.setTabGravity(TabLayout.GRAVITY_FILL);
final ViewPager viewPager = (ViewPager) getActivity().findViewById(R.id.pager);
final TemperaturePagerAdapter adapter = new TemperaturePagerAdapter
(getActivity().getSupportFragmentManager(), tabLayout.getTabCount());
viewPager.setAdapter(adapter);
viewPager.addOnPageChangeListener(new TabLayout.TabLayoutOnPageChangeListener(tabLayout));
tabLayout.setOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
});
}
}
Here is one of the Tabs/Fragments that need to display the AnyChart:
public class TemperatureFragmentTab1 extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
return inflater.inflate(R.layout.statistics_temperature_fragment_tab_1, container, false);
}
@Override
public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
AnyChartView anyChartView = Objects.requireNonNull(getActivity()).findViewById(R.id.any_chart_view_temperature_tab_1);
APIlib.getInstance().setActiveAnyChartView(anyChartView);
ProgressBar progressBar = Objects.requireNonNull(getActivity()).findViewById(R.id.loadingProgressBar_temperature_tab_1);
TextView progressText = getActivity().findViewById(R.id.loadingProgressText_temperature_tab_1);
ProgressBar progressSpinner = Objects.requireNonNull(getActivity()).findViewById(R.id.loadingProgress_temperature_tab_1);
new LongOperation(anyChartView, progressSpinner, progressBar, progressText).execute();
}
}
Here is the background task, that prepares the data and draw the AnyChart after finishing that:
public class LongOperation extends AsyncTask<Void, Integer, List<DataEntry>> {
private AnyChartView anyChartView;
private ProgressBar progressSpinner;
private ProgressBar progressBar;
private TextView progressText;
private List<Double> values;
public LongOperation(AnyChartView anyChartView, ProgressBar progressSpinner, ProgressBar progressBar, TextView progressText) {
this.anyChartView = anyChartView;
this.progressSpinner = progressSpinner;
this.progressBar = progressBar;
this.progressText = progressText;
}
@Override
protected void onPreExecute() {
progressBar.setVisibility(View.VISIBLE);
progressBar.setMax(Device.getDevices().get(0).getRecordsCount());
progressBar.setProgress(0);
progressText.setText("Loading Resources...");
progressSpinner.setVisibility(View.VISIBLE);
}
@Override
protected void onProgressUpdate(Integer... values) {
super.onProgressUpdate(values);
progressBar.setProgress(values[0]);
progressText.setText("Loading Resources: " + String.valueOf(progressBar.getProgress()) + " / " + String.valueOf(progressBar.getMax()));
}
@Override
protected List<DataEntry> doInBackground(Void... params) {
Device device = Device.getDevices().get(0);
List<DataEntry> data = new ArrayList<>();
values = new ArrayList<Double>();
for (int i=0; i < device.getRecordsCount(); i++) {
publishProgress(i);
double temperature = 0;
String timestamp = "";
try {
temperature = device.getRecord(i).getDouble("Temperature");
timestamp = device.getRecord(i).getString("Timestamp");
} catch (JSONException e) {
e.printStackTrace();
}
values.add(temperature);
data.add(new ValueDataEntry(timestamp, temperature));
}
return data;
}
@Override
protected void onPostExecute(List<DataEntry> data) {
Cartesian line = AnyChart.line();
line.animation(true);
com.anychart.core.cartesian.series.Line series = line.line(data);
series.tooltip().format("Temperatur: {%value}°C");
com.anychart.core.axismarkers.Line lineMarkerMin = line.lineMarker(0);
lineMarkerMin.value(Statistic.getMinValue(values));
com.anychart.core.axismarkers.Line lineMarkerMax = line.lineMarker(1);
lineMarkerMax.value(Statistic.getMaxValue(values));
Text textMarkerMin = line.textMarker(0);
textMarkerMin.value(Statistic.getMinValue(values));
textMarkerMin.text("Minimum: " + Double.toString(Statistic.getMinValue(values)) + "°C");
textMarkerMin.anchor("left-bottom");
textMarkerMin.align("left");
Text textMarkerMax = line.textMarker(1);
textMarkerMax.value(Statistic.getMaxValue(values));
textMarkerMax.text("Maximum: " + Double.toString(Statistic.getMaxValue(values)) + "°C");
textMarkerMax.anchor("left-bottom");
textMarkerMax.align("left");
line.xAxis(0).labels(false);
line.xAxis(0).title("Gesamte Zeit");
line.yAxis(0).title("Temperatur (in °C)");
line.draw(true);
anyChartView.setChart(line);
progressBar.setVisibility(View.INVISIBLE);
progressText.setVisibility(View.INVISIBLE);
progressSpinner.setVisibility(View.INVISIBLE);
}
}
I hope you can help me with that Problem, because I spent hours on this without any clue how to fix it.