Hello, I would like to ask a question. The version number of j2v8-debuger is 0.1.3, and the version of j2v8 is 5.0.103. I use the getOrCreateV8Debugger
and initializeWithV8DebuggerInMainThread
methods to initialize, but I always fail to debug my method, and there are breakpoints and breakpoints. The information can also be displayed in logcat
2021-01-10 16:28:04.859 15807-16174 D/ChromeDevtoolsServer: onOpen
2021-01-10 16:28:05.166 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":1,"method":"Page.canScreencast"}
2021-01-10 16:28:05.205 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":2,"method":"Page.canEmulate"}
2021-01-10 16:28:05.207 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.canEmulate
2021-01-10 16:28:05.215 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":3,"method":"Worker.canInspectWorkers"}
2021-01-10 16:28:05.227 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":4,"method":"Console.setTracingBasedTimeline","params":{"enabled":true}}
2021-01-10 16:28:05.228 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Console.setTracingBasedTimeline
2021-01-10 16:28:05.270 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":5,"method":"Console.enable"}
2021-01-10 16:28:05.276 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":6,"method":"Network.enable"}
2021-01-10 16:28:05.280 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":7,"method":"Page.enable"}
2021-01-10 16:28:05.308 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":8,"method":"Page.getResourceTree"}
2021-01-10 16:28:05.317 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":9,"method":"Debugger.enable"}
2021-01-10 16:28:05.325 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":10,"method":"Debugger.setPauseOnExceptions","params":{"state":"none"}}
2021-01-10 16:28:05.326 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Debugger.setPauseOnExceptions
2021-01-10 16:28:05.328 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":11,"method":"Debugger.setAsyncCallStackDepth","params":{"maxDepth":0}}
2021-01-10 16:28:05.329 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Debugger.setAsyncCallStackDepth
2021-01-10 16:28:05.341 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":12,"method":"Debugger.skipStackFrames","params":{"script":"","skipContentScripts":false}}
2021-01-10 16:28:05.342 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Debugger.skipStackFrames
2021-01-10 16:28:05.344 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":13,"method":"Runtime.enable"}
2021-01-10 16:28:05.345 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Runtime.enable
2021-01-10 16:28:05.348 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":14,"method":"DOM.enable"}
2021-01-10 16:28:05.399 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":15,"method":"CSS.enable"}
2021-01-10 16:28:05.410 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":16,"method":"Worker.enable"}
2021-01-10 16:28:05.411 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Worker.enable
2021-01-10 16:28:05.413 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":17,"method":"Timeline.enable"}
2021-01-10 16:28:05.413 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Timeline.enable
2021-01-10 16:28:05.425 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":18,"method":"Database.enable"}
2021-01-10 16:28:05.435 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":19,"method":"DOMStorage.enable"}
2021-01-10 16:28:05.448 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":20,"method":"Profiler.enable"}
2021-01-10 16:28:05.450 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":21,"method":"Profiler.setSamplingInterval","params":{"interval":1000}}
2021-01-10 16:28:05.453 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":22,"method":"IndexedDB.enable"}
2021-01-10 16:28:05.453 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: IndexedDB.enable
2021-01-10 16:28:05.456 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":23,"method":"Page.setShowPaintRects","params":{"result":true}}
2021-01-10 16:28:05.456 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.setShowPaintRects
2021-01-10 16:28:05.459 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":24,"method":"Page.setShowDebugBorders","params":{"show":true}}
2021-01-10 16:28:05.459 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.setShowDebugBorders
2021-01-10 16:28:05.462 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":25,"method":"Page.setShowFPSCounter","params":{"show":true}}
2021-01-10 16:28:05.463 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.setShowFPSCounter
2021-01-10 16:28:05.475 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":26,"method":"Page.setContinuousPaintingEnabled","params":{"enabled":true}}
2021-01-10 16:28:05.476 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.setContinuousPaintingEnabled
2021-01-10 16:28:05.479 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":27,"method":"Page.setShowScrollBottleneckRects","params":{"show":true}}
2021-01-10 16:28:05.479 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.setShowScrollBottleneckRects
2021-01-10 16:28:05.482 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":28,"method":"Page.getNavigationHistory"}
2021-01-10 16:28:05.483 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.getNavigationHistory
2021-01-10 16:28:05.485 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":29,"method":"Worker.setAutoconnectToWorkers","params":{"value":true}}
2021-01-10 16:28:05.486 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Worker.setAutoconnectToWorkers
2021-01-10 16:28:05.487 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":30,"method":"Inspector.enable"}
2021-01-10 16:28:05.499 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":31,"method":"Page.setShowViewportSizeOnResize","params":{"show":true,"showGrid":false}}
2021-01-10 16:28:05.501 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":32,"method":"IndexedDB.requestDatabaseNames","params":{"securityOrigin":""}}
2021-01-10 16:28:05.502 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: IndexedDB.requestDatabaseNames
2021-01-10 16:28:05.504 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":33,"method":"Page.getNavigationHistory"}
2021-01-10 16:28:05.505 15807-16174 D/ChromeDevtoolsServer: Method not implemented: Not implemented: Page.getNavigationHistory
2021-01-10 16:28:05.507 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":34,"method":"Debugger.getScriptSource","params":{"scriptId":"dynamic-action-bar-ad-v1.1015.js"}}
2021-01-10 16:28:05.533 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":35,"method":"Debugger.setBreakpointByUrl","params":{"lineNumber":468,"url":"http://app/dynamic-action-bar-ad-v1.1015.js","columnNumber":0,"condition":""}}
2021-01-10 16:28:05.611 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":36,"method":"Debugger.setBreakpointByUrl","params":{"lineNumber":107,"url":"http://app/dynamic-action-bar-ad-v1.1015.js","columnNumber":0,"condition":""}}
2021-01-10 16:28:05.631 15807-16174 V/ChromeDevtoolsServer: onMessage: message={"id":37,"method":"Debugger.setBreakpointByUrl","params":{"lineNumber":97,"url":"http://app/dynamic-action-bar-ad-v1.1015.js","columnNumber":0,"condition":""}}
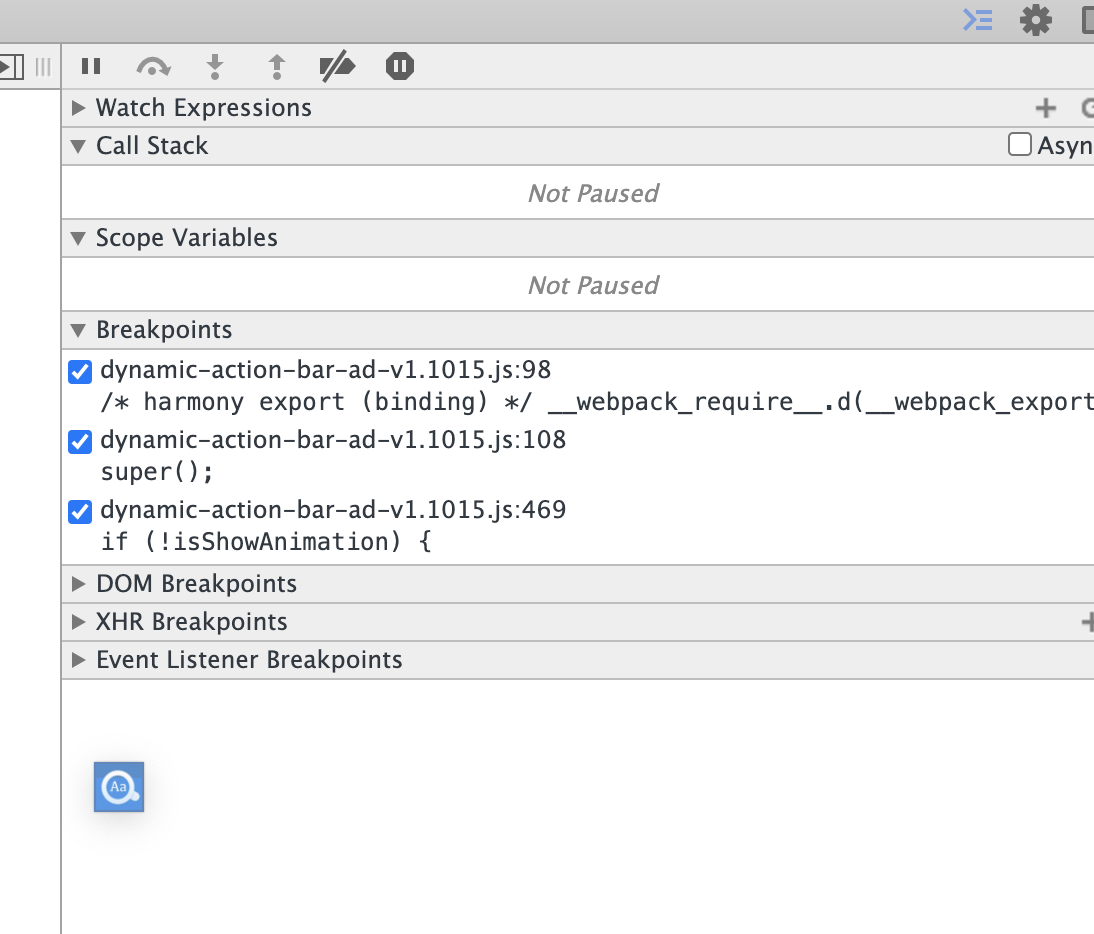
please help me